Given n
non-negative integers representing an elevation map where the width of each bar is 1
, compute how much water it can trap after raining.
Example 1:
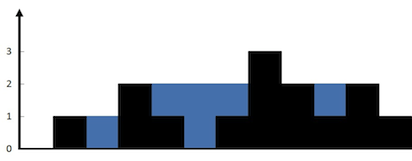
Input: height = [0,1,0,2,1,0,1,3,2,1,2,1]
Output: 6
Explanation: The above elevation map (black section) is represented by array [0,1,0,2,1,0,1,3,2,1,2,1]. In this case, 6 units of rain water (blue section) are being trapped.
Example 2:
Input: height = [4,2,0,3,2,5]
Output: 9
Constraints:
n == height.length
1 <= n <= 2 * 104
0 <= height[i] <= 105
SOLUTION:
class Solution:
def trap(self, height: List[int]) -> int:
l, r= 0, len(height) -1
maxL, maxR = height[l], height[r]
result = 0
while l < r:
if maxL < maxR:
l += 1
maxL = max(maxL, height[l])
result += maxL - height[l]
else:
r -= 1
maxR = max(maxR, height[r])
result += maxR - height[r]
return result
Time Complexity: O(n)
Space Complexity:O(1)
How it works:
- Initialize two pointers,
l
andr
, to point to the beginning and end of the elevation map, respectively. - Initialize two variables,
maxL
andmaxR
, to the height of the bars atl
andr
, respectively. - Initialize a variable
result
to 0. - While
l
is less thanr
:- If
maxL
is less thanmaxR
:- Increment
l
by 1. - Update
maxL
to the maximum ofmaxL
and the height of the bar atl
. - Add
maxL - height[l]
toresult
.
- Increment
- Otherwise:
- Decrement
r
by 1. - Update
maxR
to the maximum ofmaxR
and the height of the bar atr
. - Add
maxR - height[r]
toresult
.
- Decrement
- If
- Return
result
.
The idea behind the code is to use two pointers to scan the elevation map from left to right and right to left, respectively. The maxL
and maxR
variables keep track of the maximum height of the bars to the left of l
and to the right of r
, respectively. The result
variable keeps track of the total amount of water that can be trapped.
Comments
Post a Comment