You are given the heads of two sorted linked lists list1
and list2
.
Merge the two lists in a one sorted list. The list should be made by splicing together the nodes of the first two lists.
Return the head of the merged linked list.
Example 1:
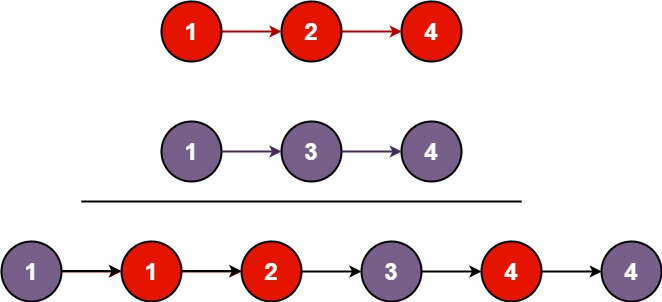
Input: list1 = [1,2,4], list2 = [1,3,4] Output: [1,1,2,3,4,4]
Example 2:
Input: list1 = [], list2 = [] Output: []
Example 3:
Input: list1 = [], list2 = [0] Output: [0]
Constraints:
- The number of nodes in both lists is in the range
[0, 50]
. -100 <= Node.val <= 100
- Both
list1
andlist2
are sorted in non-decreasing order.
SOLUTION:
- Time complexity:
The function
mergeTwoLists
takes two linked lists as input parameters:list1
andlist2
. It returns the merged sorted linked list.The initial checks
if not list1:
andif not list2:
handle the case where eitherlist1
orlist2
is empty. If one of the lists is empty, the function simply returns the other non-empty list. This is because merging an empty list with a non-empty list would result in the same non-empty list.The variables
head
andcurrent
are declared and initialized.head
is a dummy node with a value of 0, andcurrent
is used to keep track of the current position in the merged list.The condition
while list1 and list2: C
hecks if bothlist1
andlist2
are not empty. In a singly-linked list, an empty list is represented byNone
, so this condition ensures that the loop continues as long as there are still nodes to process in bothlist1
andlist2
. This loop iterates through the lists and merges the nodes based on their values.Inside the loop, the code compares the values at the current nodes of
list1
andlist2
. If the value oflist1.val
is less than or equal to the value oflist2.val
, the current node oflist1
should come first in the merged list.current.next = list1
sets thenext
attribute of thecurrent
node tolist1
, effectively appendinglist1
to the merged list.list1 = list1.next
moves thelist1
pointer to the next node inlist1
.
If the value of
list2.val
is less than the value oflist1.val
, the current node oflist2
should come first in the merged list. The process is similar to the above case but withlist2
.After setting the
next
node in the merged list,current
is updated tocurrent.next
to move the pointer to the newly added node.Once either
list1
orlist2
becomes empty, the loop terminates.If there are remaining nodes in
list1
, they are appended to the merged list by settingcurrent.next = list1
.If there are remaining nodes in
list2
, they are appended to the merged list by settingcurrent.next = list2
.Finally, the head of the merged list (excluding the dummy node) is returned as
head.next
.
Comments
Post a Comment