You are given an m x n
integer matrix matrix
with the following two properties:
- Each row is sorted in non-decreasing order.
- The first integer of each row is greater than the last integer of the previous row.
Given an integer target
, return true
if target
is in matrix
or false
otherwise.
You must write a solution in O(log(m * n))
time complexity.
Example 1:
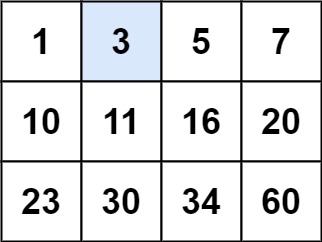
Input: matrix = [[1,3,5,7],[10,11,16,20],[23,30,34,60]], target = 3
Output: true
Example 2:
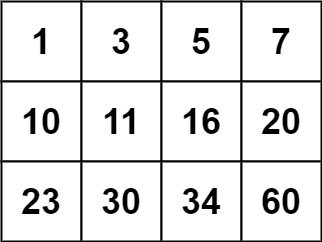
Input: matrix = [[1,3,5,7],[10,11,16,20],[23,30,34,60]], target = 13
Output: false
Constraints:
m == matrix.length
n == matrix[i].length
1 <= m, n <= 100
-104 <= matrix[i][j], target <= 104
Time Complexity: O(log m*n)
Space Complexity:O(1)
How it works:
Obtain the dimensions of the matrix:
m
represents the number of rows, andn
represents the number of columns.Initialize
firstRow
andlastRow
to track the boundaries of the search range in the matrix. SetfirstRow
as 0 (the first row) andlastRow
asm-1
(the last row).Perform a binary search to find the row where the target might be present:
- While
firstRow
is less than or equal tolastRow
, calculate the middle row asmid
using integer division. - If the target is greater than the last element in the
mid
row, updatefirstRow
tomid + 1
to search in the lower half of the remaining matrix. - If the target is less than the first element in the
mid
row, updatelastRow
tomid - 1
to search in the upper half of the remaining matrix. - If neither condition is met, it means the target might be in the
mid
row, so break the loop.
- While
Check if the search range is valid:
- If
firstRow
is greater thanlastRow
, it means the target is not present in the matrix, so returnFalse
.
- If
Calculate the actual row index,
row
, where the target might be present by taking the average offirstRow
andlastRow
.Initialize
l
andr
to track the boundaries of the search range within the chosen row. Setl
as 0 (the first column) andr
asn-1
(the last column).Perform a binary search within the chosen row to find the target value:
- While
l
is less than or equal tor
, calculate the middle column asm
using integer division. - If the target is greater than the value at
matrix[row][m]
, updatel
tom + 1
to search in the right half of the remaining row. - If the target is less than the value at
matrix[row][m]
, updater
tom - 1
to search in the left half of the remaining row. - If neither condition is met, it means the target is found at
matrix[row][m]
, so returnTrue
.
- While
If the target is not found within the chosen row, return
False
.
The code performs two binary searches: one to find the row where the target might be present and another to search for the target within that row. If the target is found, the code returns True
; otherwise, it returns False
.
Comments
Post a Comment